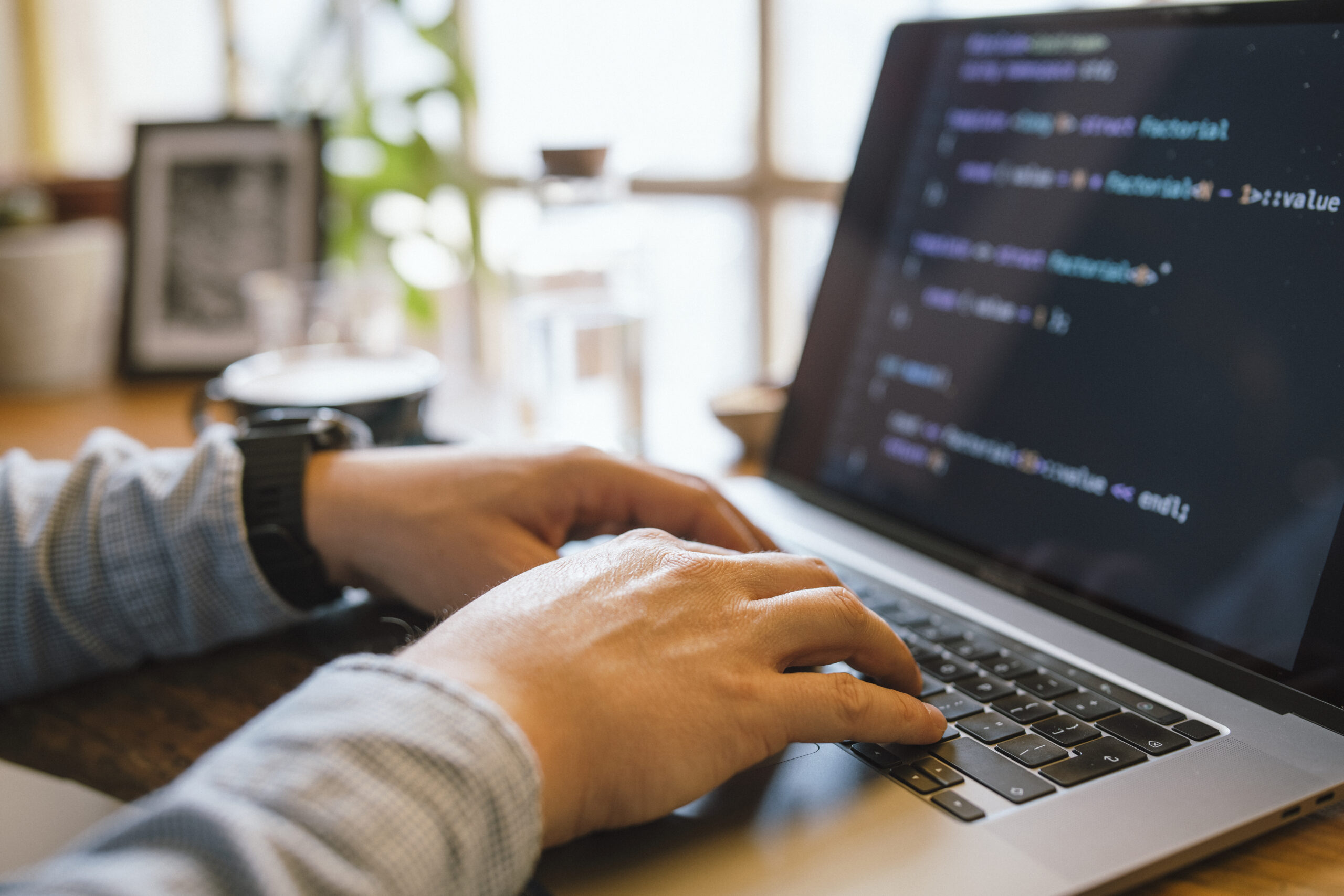
Debugging is Among the most essential — nevertheless generally missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to Believe methodically to solve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help you save several hours of annoyance and considerably transform your efficiency. Here's many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use daily. Even though creating code is 1 part of progress, being aware of the best way to interact with it correctly through execution is equally important. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these tools can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and also modify code on the fly. When utilised properly, they Enable you to observe particularly how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can transform irritating UI difficulties into workable duties.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above functioning processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation control methods like Git to grasp code heritage, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications signifies heading outside of default configurations and shortcuts — it’s about producing an personal expertise in your enhancement environment so that when problems arise, you’re not misplaced at midnight. The better you realize your resources, the more time you are able to spend solving the particular trouble rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — measures in successful debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What steps resulted in The difficulty? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you've, the easier it gets to isolate the exact ailments below which the bug takes place.
When you’ve gathered plenty of details, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions included. These checks not just support expose the problem but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t only a action — it’s a mentality. It requires patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and talk a lot more Obviously along with your staff or end users. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These concerns can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically hasten your debugging process.
Some problems are imprecise or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase through the code line by line.
A great logging method starts with realizing what to log and at what level. Typical logging ranges contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic information and facts all through growth, Information for basic occasions (like effective begin-ups), Alert for probable difficulties that don’t split the application, ERROR for precise troubles, and Deadly in the event the process can’t keep on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your technique. Concentrate on crucial activities, condition alterations, input/output values, and significant conclusion factors inside your code.
Structure your log messages Plainly and constantly. Include context, which include timestamps, request IDs, and performance names, so it’s much easier to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, smart logging is about equilibrium and clarity. Having a properly-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This mentality helps break down complicated concerns into workable sections and observe clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece collectively a clear picture of what’s happening.
Following, kind hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes recently been made to the codebase? Has this difficulty transpired ahead of below similar instances? The target is usually to slim down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem in a very controlled atmosphere. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Shell out close awareness to little aspects. Bugs typically hide from the least envisioned areas—similar to a lacking semicolon, an off-by-one mistake, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Lastly, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Assessments
Producing checks is among the most effective approaches to transform your debugging competencies and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-confidence when earning variations to your codebase. A nicely-tested application is easier to debug because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably lessening enough time expended debugging. Device exams are Specifically beneficial for catching regression bugs—concerns that reappear right after Formerly being fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that various aspects of your application function alongside one another efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails consistently, it is possible to focus on fixing the bug and look at your test pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from the irritating guessing match right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult situation, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option just after Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious do the job while in the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You might quickly discover a lacking semicolon, a logic flaw, or maybe website a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it surely really brings about quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to improve to be a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can educate you anything important if you take some time to mirror and assess what went Erroneous.
Get started by asking yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The responses normally expose blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital parts of your progress journey. In any case, some of the ideal developers will not be those who compose perfect code, but individuals who continuously understand from their mistakes.
Ultimately, each bug you resolve provides a fresh layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.